Template Engine
Overview
iWorkflow contains a template engine, similar to other workflow tools. Templates are also sometimes referred to as expressions or functions.
Templates allow you to insert dynamic data into your workflow actions, and even reference data that was output from previous actions.
Templatable Fields
It’s easy to identify a field that supports templates. Templatable fields have a blue gradient border around them and a different (monospace) font compared to a non-templatable field.
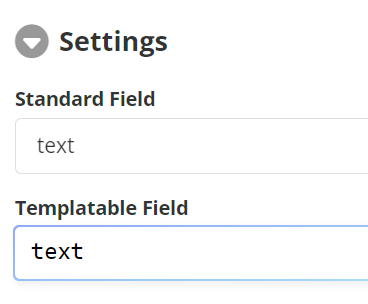
An example of a standard (non-templatable) versus a templatable field.
Templates are Optional
Any field that can be templated does not have to be templated. The template language only recognizes text between {{
… }}
or {%
… %}
as template code. Anything not surrounded by those tags is treated as-is.
For example, on the HTTP action, the Method property is templatable, but you can just enter the word GET or POST directly - it does not have to be a template.
Template Language
iWorkflow uses a derivative of the Liquid template language. You can read a more in-depth introduction to the Liquid template language here.
We’ll cover the basics necessary for iWorkflow below.
Objects and Fields
Liquid evaluates and replaces any text inside {{
and }}
. So, if you have a variable called greeting
that was set to the value “Hi there”, you could write the following template and get this output:
Template | Output |
---|---|
|
|
Filters and Functions
Functions or methods in other languages are referred to as filters in Liquid. Filters can be used for a variety of things, such as converting a string to uppercase, formatting a date, parsing JSON, and lots more.
Filters are called by adding a pipe character (|
) between the field and the filter. For example:
Template | Output |
---|---|
|
|
Filters also support parameters - for example, the join
filter joins an array together into a string and takes the delimiter as a parameter. So if you enter:
Template | Output |
---|---|
|
|
Data Types
Liquid supports the same data types that JSON does. You can use the type
filter to get the data type name of an expression or variable.
iWorkflow also contains some special cases to work with JSON objects. In these cases, the data types will be JObject
, JArray
, etc.
Here are the supported data types for iWorkflow:
Template | Output |
---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Whitespace
When writing templates, whitespace is generally preserved outside of the curly braces. By contrast, any whitespace contained between {{
and }}
is generally ignored.
In the following table, the · symbol will represent an invisible space character.
Template | Output |
---|---|
|
|
|
|
|
|
msg = |
|
These examples show that anything outside of {{...}}
is preserved, which can potentially add unwanted whitespace to your data.
Practical Examples
Referencing Data
Many actions will ask for an array or dataset as input. To provide the dataset, first find the { } Output Property from the previous action. The name you enter in the Output Property becomes a property on the input object.
For example, if you have a Fetch IQA Data action that is set to write an output property called memberDataset
, then in an action below that, such as the Delta Hash action, you can provide the array as an input by providing this template expression:
{{ input.memberDataset }}
File Names
When using the FTP or SFTP actions, for example, you may need to create a random or date-based filename. You can achieve this with templates. For example:
// Randomized filename
my-test-file-{{ guid }}.csv
// Date-based filename
my-date-file-{{ date.now | format 'yyyy-MM-dd' }}.csv
Changing Data Types
If you have a dataset containing data in an incorrect format, for example numbers encased in strings ("100"
), you can use the https://csiinc.atlassian.net/wiki/spaces/iDMS/pages/111378454 action in conjunction with the template engine to change the data type.
For example, if you had a dataset with a property called count
that you wanted to convert from a string to a number, you could use the following select expression to parse the number as an integer:
{{ item.count | to_int }}
Your dataset would be altered like this:
Before
[
{ "count": "100" },
{ "count": "250" },
{ "count": "500" }
]
After
[
{ "count": 100 },
{ "count": 250 },
{ "count": 500 }
]